Boilerplate project using Ionic & angularJS for NodeJS running on Bluemix
So let's break this down. We need to create a Web application that we can view on a desktop/laptop web-browser or a Tablet or a mobile device. We don't need it to be running anything native to a mobile device, good old HTML5 with some JavaScript libraries will be good enough for the task.
This Boilerplate will be using the following technologies:
I'm going to assume that you know something about each of these, if not Google/Bing/DuckDuckGo are you friends.
The objective is to create a simple framework application that we can put into place that does some of the basic functionality, allowing us to then build out and do the custom complex parts, which is where we really want to focus our time and effort.
For this, we shall use a basic layout like this:
Where the left-hand side menu will open/close if the user either swipes it in/out (or presses the hamburger icon).
The View section will load the view .html files accordingly and the button on each view will load the next required view .html and controller. The controller code has the ability to then be extended to call REST API services to gather data, reformat if necessary and output to objects that are then rendered in the view .html file content.
I shall re-use the same format/structure for all of the Boilerplates for consistency.
Select a NodeJS SDK (even though we will throw away the default info that is created)
This name is just for screenshot purposes - the real name is slightly different from this, as you'll see later:
The first step is to make sure you have the CF CLI installed (I would have thought you would have done so by now?), also you will have already installed NODEJS and NPM (you'll need these later):
You can download the starter code .zip, but, we'll be replacing it with our source-code, it won't do any harm to download it, just to see what files we have changed.
Then you must do this part and this is custom to your Bluemix account. Replace this values shown here with your values.
Don't do the 'cf push' command just yet.
Now that you have downloaded the code, you can follow the instructions above,
>cd boilerplateIonicAngularJS
Now, I break the rules a bit here as I've included the node_modules folder in the source-code, "best practice" is that you do not do this, you specify the modules you want to use in the package.json file:
Here we are telling the project that we need these resources, in the dependencies section.
As these files can be rather large, I can see the logic that says to not pass them around but to download the fresh/latest version for each project. That said, you need to have them, so you'll execute a command that will read the package.json file and download the required files into the node_modules folder:
>npm install
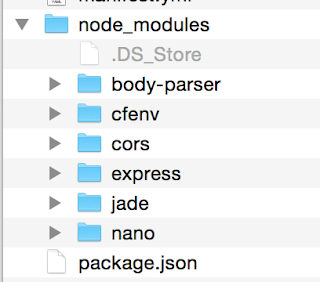
Minor word of caution, if you originally included a node_module and then decided to remove it, the folder will still stay there. You can see this above, with the 'jade' folder. This is no longer needed. If you were doing a fresh 'npm install', you wouldn't get this folder, but just be aware of this in the future.
You will also note that we define in the package.json file that we want Bluemix to run 'node app.js' to start the NodeJS application after we have uploaded it, or, stop / started it on Bluemix.
All done. Now, the above is all standard stuff that you would need to do as the basics for any NodeJS application. So, there should not be anything new here, I'm just clarifying the steps. If this took longer than 5 minutes to do, then you need to upgrade your broadband and/or stop checking facebook.
The folder structure is like so:
The index.html file above is the mainpage of the web site.
The /scripts/app.js file is the AngularJS routing file (NOT to be mixed up with the app.js that is executed on the Bluemix server via node)
The /scripts.app.js file contains the references to the /controllers/*.js files and the matching /views/*.html file.
For instance, when the user clicks on a button on the mainpage.html, the controller code in mainpage.js will then navigate the user to 'page2.html'.
This means that page2.html will be rendered on the web page and the page2.js controller will be executed and will be listening for events coded into it.
The above code now matches the required boilerplate simple layout structure.
This will upload all of the files in your folder to the Bluemix application space.
The first time you do this, you will want to upload everything.
The second time onwards, you probably do not need to upload the node_modules folder as nothing should have changed and there are a lot of files being copied for no real value.
You can create a file on the top level folder and name it .cfignore and insert this one line:
node_modules
This nifty little trick means that when you perform a 'cf push', it will upload all the files except the node_modules folder. Saving time and bandwidth.
Once you have uploaded the folder, you should see that you application has started and the URL is ready to be accessed:
Visit the URL in a web browser and the app is running. Swiping brings out the menu and pressing the button on each view takes you to the next view/screen.
As mentioned, this is a boilerplate simple framework application that you can use to then build upwards from. It will have saved you a fair amount of time, as it is all proven to work, all references are valid. You just now need to extend the controller code as you wish and obviously make the UI look a lot nicer than it does now.
This Boilerplate will be using the following technologies:
- HTML5
- Ionic
- AngularJS
- NodeJS running on Bluemix
I'm going to assume that you know something about each of these, if not Google/Bing/DuckDuckGo are you friends.
The objective is to create a simple framework application that we can put into place that does some of the basic functionality, allowing us to then build out and do the custom complex parts, which is where we really want to focus our time and effort.
For this, we shall use a basic layout like this:
Where the left-hand side menu will open/close if the user either swipes it in/out (or presses the hamburger icon).
The View section will load the view .html files accordingly and the button on each view will load the next required view .html and controller. The controller code has the ability to then be extended to call REST API services to gather data, reformat if necessary and output to objects that are then rendered in the view .html file content.
I shall re-use the same format/structure for all of the Boilerplates for consistency.
Setting up Bluemix
First things first, we need to create a new application in Bluemix. All standard stuff.Select a NodeJS SDK (even though we will throw away the default info that is created)
This name is just for screenshot purposes - the real name is slightly different from this, as you'll see later:
The first step is to make sure you have the CF CLI installed (I would have thought you would have done so by now?), also you will have already installed NODEJS and NPM (you'll need these later):
You can download the starter code .zip, but, we'll be replacing it with our source-code, it won't do any harm to download it, just to see what files we have changed.
Then you must do this part and this is custom to your Bluemix account. Replace this values shown here with your values.
Don't do the 'cf push' command just yet.
Now that you have downloaded the code, you can follow the instructions above,
>cd boilerplateIonicAngularJS
Now, I break the rules a bit here as I've included the node_modules folder in the source-code, "best practice" is that you do not do this, you specify the modules you want to use in the package.json file:
Here we are telling the project that we need these resources, in the dependencies section.
As these files can be rather large, I can see the logic that says to not pass them around but to download the fresh/latest version for each project. That said, you need to have them, so you'll execute a command that will read the package.json file and download the required files into the node_modules folder:
>npm install
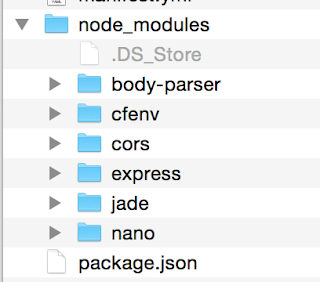
Minor word of caution, if you originally included a node_module and then decided to remove it, the folder will still stay there. You can see this above, with the 'jade' folder. This is no longer needed. If you were doing a fresh 'npm install', you wouldn't get this folder, but just be aware of this in the future.
You will also note that we define in the package.json file that we want Bluemix to run 'node app.js' to start the NodeJS application after we have uploaded it, or, stop / started it on Bluemix.
All done. Now, the above is all standard stuff that you would need to do as the basics for any NodeJS application. So, there should not be anything new here, I'm just clarifying the steps. If this took longer than 5 minutes to do, then you need to upgrade your broadband and/or stop checking facebook.
Web Application architecture
As this is a boilerplate foundation application, all we need it to do is to have an index.html page that links to the right JavaScript libraries, a folder structure setup to contain the web pages (views) and the AngularJS routing setup so that when a request is made to go to a specific page it is routed correctly and the correct AngularJS controller is called.The folder structure is like so:
The index.html file above is the mainpage of the web site.
The /scripts/app.js file is the AngularJS routing file (NOT to be mixed up with the app.js that is executed on the Bluemix server via node)
The /scripts.app.js file contains the references to the /controllers/*.js files and the matching /views/*.html file.
For instance, when the user clicks on a button on the mainpage.html, the controller code in mainpage.js will then navigate the user to 'page2.html'.
This means that page2.html will be rendered on the web page and the page2.js controller will be executed and will be listening for events coded into it.
The above code now matches the required boilerplate simple layout structure.
Upload the content to Bluemix
Now, we can perform the 'cf push' as mentioned earlier.This will upload all of the files in your folder to the Bluemix application space.
The first time you do this, you will want to upload everything.
The second time onwards, you probably do not need to upload the node_modules folder as nothing should have changed and there are a lot of files being copied for no real value.
You can create a file on the top level folder and name it .cfignore and insert this one line:
node_modules
This nifty little trick means that when you perform a 'cf push', it will upload all the files except the node_modules folder. Saving time and bandwidth.
Once you have uploaded the folder, you should see that you application has started and the URL is ready to be accessed:
Visit the URL in a web browser and the app is running. Swiping brings out the menu and pressing the button on each view takes you to the next view/screen.
As mentioned, this is a boilerplate simple framework application that you can use to then build upwards from. It will have saved you a fair amount of time, as it is all proven to work, all references are valid. You just now need to extend the controller code as you wish and obviously make the UI look a lot nicer than it does now.
Comments
Post a Comment